Introduction
Good morning, everyone! It’s wonderful to see you all here, ready to delve deeper into the exciting world of LoRa technology and mesh networking. Over the past few lectures, we’ve explored advanced LoRa features, implemented secure communication, and learned about multi-hop routing and data aggregation. Today, we’re going to bring all those concepts together by **implementing a mesh network using LilyGO devices**.
Imagine you’re tasked with deploying a network of sensors across a mountainous region to monitor environmental conditions. The terrain is challenging, and direct communication between distant nodes is impossible due to obstacles and range limitations. A mesh network offers a solution, allowing nodes to relay data through intermediate devices, ensuring reliable communication across the entire area.
By the end of today’s lecture, you’ll have a step-by-step understanding of how to create a basic mesh network with LilyGO devices. We’ll delve into data routing, node communication, and the practical aspects of setting up and testing your network. This hands-on approach will empower you to design and deploy mesh networks tailored to various real-world applications.
So, let’s embark on this journey and unlock the full potential of our LilyGO devices in building resilient and scalable mesh networks.
Section 1: Revisiting Mesh Networking Concepts
Before we get into the practical steps, let’s briefly revisit the fundamental concepts of mesh networking to ensure we’re all on the same page.
What is a Mesh Network?
A mesh network is a decentralized network topology where each node is interconnected with others, directly or indirectly. This structure allows data to be transmitted from one node to any other node in the network through multiple pathways.
Key Characteristics:
– Reliability: If one node fails or a connection is lost, the network can reroute data through alternative paths.
– Scalability: New nodes can be added without significant reconfiguration.
– Flexibility: Nodes can be moved or rearranged with minimal impact on the network.
Why Use Mesh Networks with LoRa?
– Extended Range: Overcome the limitations of individual node range by relaying data through multiple nodes.
– Robust Communication: Enhance reliability in challenging environments with obstacles or interference.
– Energy Efficiency: Nodes can operate at lower transmission power, conserving energy while maintaining communication.
Section 2: Planning Your Mesh Network
Effective implementation begins with careful planning. Let’s consider the essential aspects you need to address before setting up your mesh network.
1. Network Topology Design
– Determine Node Placement: Assess the geographic area and decide where to place each node for optimal coverage.
– Identify Roles: Assign specific roles to nodes, such as sensor nodes, relay nodes, and gateway nodes.
– Consider Redundancy: Plan for multiple pathways to ensure reliability in case of node failures.
2. Hardware Selection
– Choose Appropriate Devices: LilyGO T-Beam and T-Deck are excellent choices due to their built-in LoRa modules and processing capabilities.
– Ensure Compatibility: All devices should be compatible with the chosen networking protocols and libraries.
3. Software and Libraries
– Select Networking Libraries: Decide on the libraries that will manage the mesh networking functions. Popular choices include the RadioHead library and ESP-MESH.
– Plan for Future Expansion: Choose software solutions that allow scalability and future enhancements.
4. Power Management
– Power Sources: Decide whether nodes will be battery-powered, solar-powered, or connected to a permanent power source.
– Energy Efficiency: Implement strategies to conserve power, such as sleep modes or duty cycling.
5. Security Considerations
– Encryption: Plan how to secure your data transmissions using encryption methods.
– Authentication: Ensure that only authorized nodes can join and communicate within the network.
Section 3: Setting Up the Development Environment
Before we start coding, let’s set up our development environment to ensure a smooth implementation process.
1. Install the Arduino IDE
– Download and Install: Ensure you have the latest version of the Arduino IDE installed on your computer.
– Configure for ESP32: Add the ESP32 board manager URL to your Arduino IDE preferences and install the ESP32 boards.
2. Install Necessary Libraries
– RadioHead Library: This library provides a comprehensive suite for wireless communication and supports mesh networking.
– Other Dependencies: Install any additional libraries required for your specific application, such as sensor libraries.
3. Gather Your Hardware
– LilyGO Devices: Have all your devices ready and ensure they are functioning correctly.
– Cables and Antennas: Prepare USB cables for programming and LoRa antennas for each device.
Section 4: Understanding Data Routing and Node Communication
Let’s delve into how data routing and node communication work within a mesh network.
Data Routing Mechanisms
– Routing Tables: Each node maintains a table of known routes to other nodes.
– Route Discovery: Nodes discover routes by broadcasting requests and receiving replies.
– Hop Count: Data packets include a hop count to prevent infinite loops and manage the number of times a packet is forwarded.
Node Communication Protocols
– Packet Structure: Define a consistent packet format that includes source, destination, payload, and any necessary headers.
– Acknowledgments: Implement acknowledgment packets to confirm successful data transmission.
– Error Handling: Include mechanisms to handle lost or corrupted packets, such as retries or alternative routing.
Challenges in Mesh Networks
– Latency: Data may take longer to reach its destination due to multiple hops.
– Bandwidth Limitations: The network’s capacity can be affected by the number of nodes and traffic volume.
– Synchronization: Keeping nodes synchronized is essential to avoid collisions and manage power consumption.
Section 5: Step-by-Step Guide to Creating a Basic Mesh Network
Now, let’s get hands-on and start building our mesh network.
Scenario: We’ll create a simple mesh network with three nodes:
– Node 1 (Sensor Node): Collects data and sends it to the network.
– Node 2 (Relay Node): Forwards data between nodes.
– Node 3 (Gateway Node): Receives data and processes it, possibly sending it to a server or displaying it.
Step 1: Assign Unique IDs to Each Node
Assign a unique identifier to each node to distinguish them within the network.
– Node 1 ID: 101
– Node 2 ID: 102
– Node 3 ID: 103
Step 2: Initialize the Nodes
For each node, set up the necessary initialization in your code.
Initialization Includes:
– Setting Up LoRa Communication: Configure frequency, bandwidth, and other LoRa settings.
– Initializing Mesh Networking Library: Initialize the mesh library with the node’s unique ID.
Step 3: Implementing Node Functions
Node 1 (Sensor Node):
– Data Collection: Read data from sensors, such as temperature or humidity.
– Data Transmission: Send the collected data to the gateway node via the mesh network.
– Handling Acknowledgments: Wait for confirmation that the data was received.
Node 2 (Relay Node):
– Routing Logic: Receive data packets and determine the next hop towards the destination.
– Forwarding Data: Transmit data packets to the appropriate node.
– Maintaining Routing Tables: Update routes based on network changes.
Node 3 (Gateway Node):
– Data Reception: Receive data packets from sensor nodes.
– Data Processing: Interpret and process the received data.
– Optional Actions: Display data on a screen, store it locally, or forward it to a cloud server.
Step 4: Testing Communication Between Nodes
– Initial Tests: Start by testing communication between two nodes to ensure basic functionality.
– Scaling Up: Add the third node and test multi-hop communication.
– Monitoring Outputs: Use serial monitors to observe data transmissions and receptions.
Step 5: Implementing Error Handling and Retries
– Acknowledgments: Ensure that nodes send acknowledgments upon receiving data.
– Retries: Implement retry mechanisms if acknowledgments are not received within a certain timeframe.
– Timeouts: Set appropriate timeouts to prevent indefinite waiting periods.
Section 6: Practical Tips for Successful Implementation
1. Start Simple
– Begin with Direct Communication: Test sending and receiving data between two nodes without the mesh network.
– Gradually Introduce Complexity: Add mesh networking features step by step.
2. Consistent Packet Formats
– Define a Standard Format: Ensure all nodes interpret data packets correctly by using a consistent structure.
– Include Essential Information: Source ID, destination ID, payload length, and payload data.
3. Debugging Techniques
– Serial Monitoring: Use serial output to print debug messages and monitor node behavior.
– LED Indicators: Utilize LEDs to provide visual feedback for events like data transmission or errors.
4. Managing Power Consumption
– Optimize Transmission Power: Set the minimum required transmission power to conserve energy.
– Sleep Modes: Implement sleep modes for nodes when they are idle.
5. Document Your Code
– Comments: Include comments in your code to explain complex logic.
– Version Control: Use a version control system like Git to track changes and collaborate.
Section 7: Understanding Node Communication in Depth
Let’s explore how nodes communicate within the mesh network at a deeper level.
Routing Protocols
– Flooding: A simple method where nodes broadcast messages to all neighbors. Not efficient for large networks due to redundancy.
– Proactive Routing: Nodes maintain up-to-date routes to all other nodes, which can consume resources.
– Reactive Routing: Routes are discovered on-demand, reducing overhead but potentially increasing latency.
Implementing a Routing Algorithm
– Choose an Algorithm: Select one that balances efficiency and resource consumption for your network size.
– Routing Tables: Maintain a table of known routes with metrics like hop count or signal strength.
– Route Maintenance: Update routes periodically or when changes are detected.
Data Packet Lifecycle
1. Creation: The source node creates a data packet with the destination ID and payload.
2. Transmission: The packet is sent to neighboring nodes.
3. Forwarding: Intermediate nodes check if they are the destination; if not, they forward the packet.
4. Reception: The destination node receives and processes the packet.
5. Acknowledgment: An acknowledgment packet is sent back to the source following the reverse path.
Section 8: Enhancing the Mesh Network
Once the basic mesh network is operational, you can enhance it with additional features.
1. Implementing Security Measures
– Encryption: Protect data using encryption methods to prevent eavesdropping.
– Authentication: Ensure that only authorized nodes can participate in the network.
2. Adding More Nodes
– Scalability Testing: Add more nodes to test how the network handles increased size.
– Load Balancing: Distribute traffic evenly to prevent overloading certain nodes.
3. Integrating with Cloud Services
– Data Upload: Configure the gateway node to send data to cloud platforms like AWS IoT or Google Cloud.
– Remote Monitoring: Enable remote access to monitor and manage the network.
4. Implementing Over-the-Air Updates
– Firmware Updates: Allow nodes to receive updates wirelessly, reducing the need for physical access.
– Security Considerations: Ensure updates are secure to prevent unauthorized code execution.
Section 9: Troubleshooting Common Issues
Even with careful planning, you may encounter challenges. Here are some common issues and how to address them.
1. Nodes Not Communicating
– Check LoRa Settings: Ensure frequency, bandwidth, and other settings match across nodes.
– Verify IDs: Confirm that node IDs are unique and correctly assigned.
– Signal Interference: Look for sources of interference or adjust node placement.
2. High Latency or Packet Loss
– Optimize Routing: Evaluate the routing algorithm for efficiency.
– Adjust Power Levels: Increase transmission power if signal strength is weak.
– Manage Traffic: Implement mechanisms to prevent network congestion.
3. Nodes Not Joining the Network
– Authentication Errors: Check security settings if authentication is implemented.
– Firmware Compatibility: Ensure all nodes are running compatible firmware versions.
4. Unexpected Power Consumption
– Sleep Modes: Verify that sleep modes are correctly implemented and activated.
– Peripherals: Disable unused peripherals that may consume power.
Section 10: Real-World Applications and Case Studies
To solidify your understanding, let’s look at some real-world examples where mesh networks with LilyGO devices have been successfully implemented.
Case Study 1: Environmental Monitoring in Remote Areas
– Challenge: Collecting data from sensors spread across a remote forest with no cellular coverage.
– Solution: Deploy a mesh network of LilyGO devices to relay data from sensors back to a central node connected to a satellite uplink.
– Outcome: Achieved reliable data collection over vast distances without the need for physical retrieval.
Case Study 2: Smart Agriculture
– Challenge: Monitoring soil moisture, temperature, and humidity across large farms.
– Solution: Implemented a mesh network where sensor nodes report data to gateway nodes, which then make irrigation decisions or notify farmers.
– Outcome: Improved crop yields and water usage efficiency through real-time monitoring.
Case Study 3: Emergency Communication Networks
– Challenge: Establishing communication in disaster-stricken areas where infrastructure is damaged.
– Solution: Set up a mesh network to provide basic communication capabilities for rescue teams and affected populations.
– Outcome: Enabled coordination of rescue efforts and improved response times.
Conclusion
Today, we’ve taken a comprehensive journey through the process of implementing a mesh network with LilyGO devices. By understanding the principles of mesh networking, planning effectively, and following a step-by-step approach, you’re now equipped to build and deploy your own mesh networks for various applications.
Mesh networking unlocks tremendous potential in expanding the reach and resilience of communication systems, especially in challenging environments or large-scale deployments. As you apply these concepts, remember to consider the specific needs of your application, optimize for efficiency, and prioritize security.
I encourage you to continue experimenting, refining your networks, and exploring advanced features that can further enhance your systems. The knowledge and skills you’ve gained not only broaden your technical capabilities but also open doors to innovative solutions that can make a real difference in the world.
Questions and Discussion
Let’s take some time to address any questions or insights you might have.
Question: How can we ensure the mesh network remains reliable as we add more nodes?
Answer: As you scale up, it’s important to monitor network performance and adjust accordingly. Implement efficient routing protocols that can handle increased traffic, optimize node placement to prevent bottlenecks, and consider hierarchical network structures to manage complexity. Regularly testing and analyzing network metrics can help identify and resolve issues proactively.
Question: What are some strategies to reduce power consumption in relay nodes that need to be active more frequently?
Answer: Implementing duty cycling where nodes are only active when necessary can help. Using energy-efficient hardware, optimizing transmission power, and leveraging energy-harvesting methods like solar power are effective strategies. Additionally, intelligent routing that minimizes unnecessary transmissions can conserve energy.
Question: Can different types of devices be integrated into the same mesh network?
Answer: Yes, as long as the devices are compatible with the communication protocols and can be programmed to understand the network’s data formats and routing mechanisms. This allows for flexible networks where various sensors and actuators can work together seamlessly.
Additional Resources
– Mesh Networking Protocols: Explore protocols like Zigbee or Thread for alternative approaches to mesh networking.
– LilyGO Community Forums: Engage with other developers to share experiences and solutions.
– Advanced Mesh Networking Techniques: Research topics like self-healing networks and adaptive routing algorithms.
Closing Remarks
As we wrap up today’s lecture, I want to commend you all for your dedication and enthusiasm. Implementing a mesh network is a significant achievement that combines theory, practical skills, and creative problem-solving. The applications of this technology are vast and impactful, from environmental conservation to enhancing connectivity in underserved areas.
In our next lecture, we’ll focus on optimizing network performance and reliability, diving deeper into advanced techniques to fine-tune your mesh networks. We’ll explore signal strength optimization, power management, and strategies to ensure your networks operate at their best under various conditions.
Thank you for your active participation today. I’m excited to see how you’ll apply these concepts in your projects and the innovative solutions you’ll develop.
See you all in the next lecture!
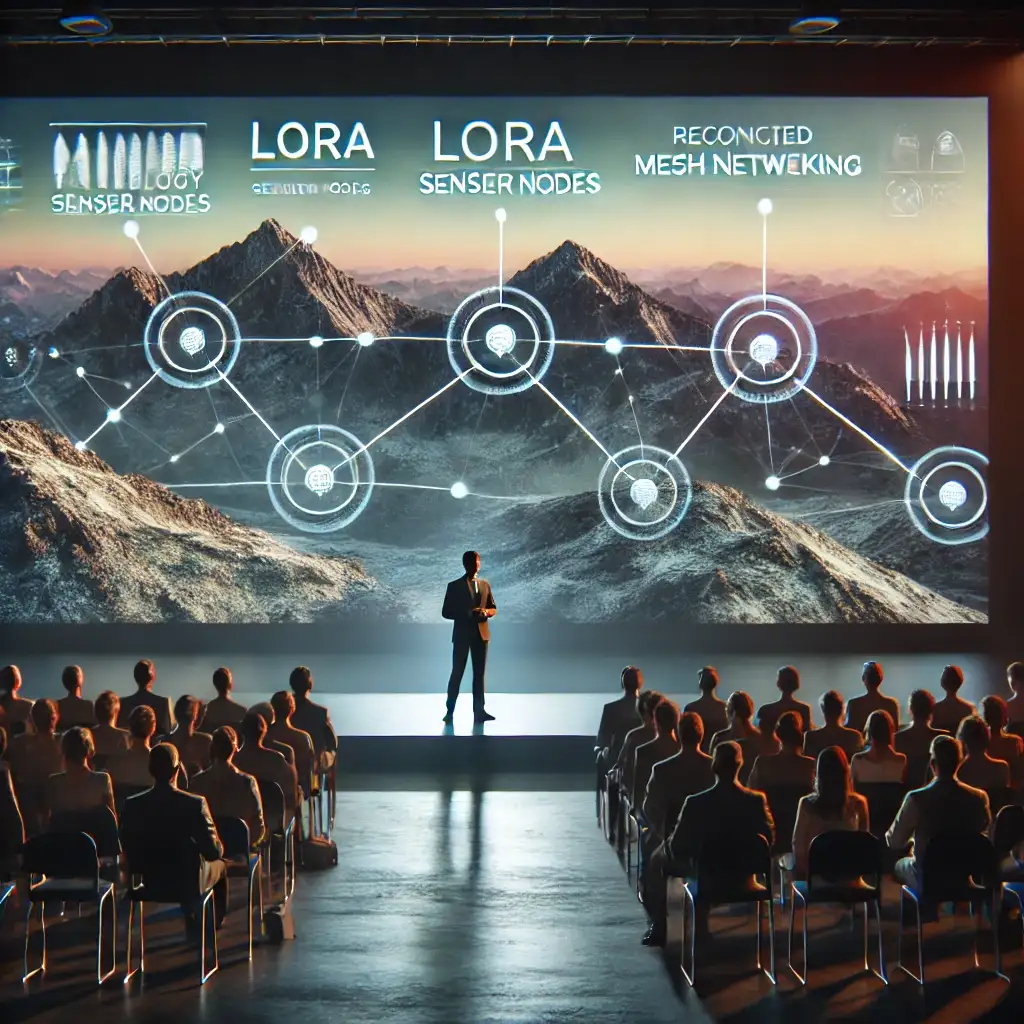
To see our Donate Page, click https://skillsgaptrainer.com/donate
To see our Instagram Channel, click https://www.instagram.com/skillsgaptrainer/
To see some of our Udemy Courses, click SGT Udemy Page
To see our YouTube Channel, click https://www.youtube.com/@skillsgaptrainer